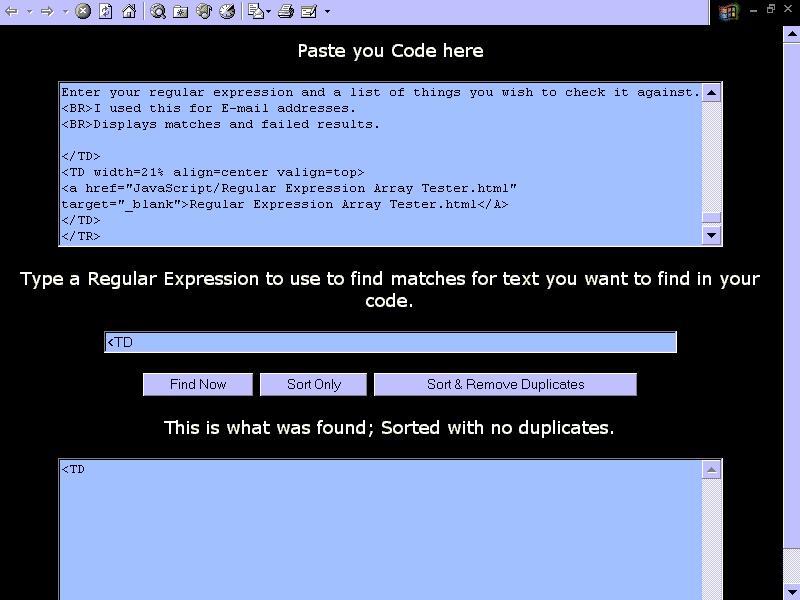
One of the most important set of tools across programming languages, the command line, and computer science in general are Regular Expressions. Regular Expressions, (or Regex / Regexp for short) is a defined syntax for search patterns and looking up specific words, phrases or sequences of characters in a file. While they look quite convoluted and complex to the naked eye, the basic formulas and rules for working with regular expression patterns are fairly simple, and extremely powerful once mastered. This post will give an introduction to the basic syntax of regex as applied to the JavaScript language, although the syntax is virtually universal across all programming languages. When in doubt, you can check the syntax of your regex patterns using an application such as regex101.com.
The basic search pattern
Any pattern that you want to search for will be placed inside two forward slash marks /:
let searchPattern = /my/;
JavaScript has a .test() method that can be applied to a variable, returning true if the pattern is found in the variable .test() is applied to, returning false if it is not. The regex you want to search for will be passed to the .test() method:
let testPhrase = “This is my book”;
let searchPattern = /my/;
testPhrase.test(searchPattern);
// Returns true
On its own, search patterns are case sensitive:
let testPhrase = “My book is over there”;
let searchPattern = /my/;
testPhrase.test(searchPattern);
// Returns false
To make a search case-insensitive, we can append the i flag after the last forward slash in the regex:
let testPhrase = “My book is over there”;
let searchPattern = /my/i;
testPhrase.test(searchPattern);
// Returns true
Returning matches
.test() is useful for checking for the existence of a pattern, but it’s somewhat limited. Especially in instances when there are multiple occurrences of a patterns in a search, you might want to see a result of all the matches found. We can use the .match() function for this to return all matches.
First, however, we will need to apply the search globally. We can do this by adding the g flag in the same way we added the i flag. Both flags can be used in tandem or individually:
let testPhrase = “My book is over there, but that one is not my book.”;
let searchPattern = /my/gi;
testPhrase.match(searchPattern);
// Returns [“My”, “my”];
.match() will return all results found in an array, as in the above example.
Using a wildcard search
In instances where you only know a portion of what you’re searching for, you can use a wildcard search, supplying the characters you know will be in the match, appended or prepended with the wildcard symbol, .:
let testPhrase1 = “He is tall.”;
let testPhrase2 = “I couldn’t tell.”;
let searchPattern = /t.ll/gi;
testPhrase1.match(searchPattern);
// Returns [“tall”];
testPhrase2.match(searchPattern);
// Returns [“tell”];
Specifying multiple possible characters
Similar to a wildcard but with a more narrowed, search, we can specify a set of characters as a variable option in our search. Any of the characters that could be included in a valid search would be placed inside brackets ([ and ]):
let testPhrase1 = “ted”;
let testPhrase2 = “tod”;
let testPhrase3 = “tad”;
let searchPattern = /t[aeo]d/gi;
testPhrase1.match(searchPattern);
// Returns [“ted”];
testPhrase2.match(searchPattern);
// Returns [“tod”];
testPhrase3.match(searchPattern);
// Returns [“tad”];
Conclusion
We’ve just scratched the surface of what regular expressions can offer, but they are immensely prevalent throughout computer science and programming practices. When used effectively, they simplify searching for files on the command line, as well as provide complete precision over match results in an application or simple script.
For more information on web design or app development services in Los Angeles, consult an expert at Sunlight Media LLC.